The segmentation fault, also known as segfault, is a type of computer error that occurs whenever an unexpected condition causes the processor to attempt to access a memory location that's outside its own program storage area. The term “segmentation” refers to a memory protection mechanism used in virtual memory operating systems.
This specific error arises because data is typically shared on a system using different memory sections, and the program storage space is shared among applications.
Segmentation faults are usually triggered by an access violation, which occurs when the CPU attempts to execute instructions outside its memory area or tries to read or write into some reserved address that does not exist. This action results in halting the current application and generates an output known as Segmentation Fault.
#1. What are the Symptoms of Segmentation Fault?
The symptoms of segmentation faults may vary depending on how and where they're generated. Typically, this error is generated due to one of the following conditions:
#a. Dereferencing a null pointer
Programming languages offer references, which are pointers that identify where in memory an item is located. A null pointer is a special pointer that doesn't point to any valid memory location. Dereferencing (accessing) null pointer results in segmentation faults or null pointer exceptions.
/**
* @file main.c
* @author freecoder
* @brief this program allow to handle a segmentation fault error
*
* @version 1.0
* @date 8 Jan. 2022
*
* @copyright Copyright (c) 2022
*
*/
#include <stdio.h>
/* main program entry */
int main(int argc, char **argv)
{
/* local variables */
unsigned int *puiPointer = NULL;
/* body program */
*puiPointer = 20;
return 0;
}
after compiling and running the program with the gdb command, the segmentation fault error appears:
➜ Article-XX gcc -g main.c -o main
➜ Article-XX ./main
[1] 7825 segmentation fault ./main
➜ Article-XX gdb ./main
GNU gdb (Debian 10.1-1.7) 10.1.90.20210103-git
Copyright (C) 2021 Free Software Foundation, Inc.
License GPLv3+: GNU GPL version 3 or later <http://gnu.org/licenses/gpl.html>
This is free software: you are free to change and redistribute it.
There is NO WARRANTY, to the extent permitted by law.
Type "show copying" and "show warranty" for details.
This GDB was configured as "x86_64-linux-gnu".
Type "show configuration" for configuration details.
For bug reporting instructions, please see:
<https://www.gnu.org/software/gdb/bugs/>.
Find the GDB manual and other documentation resources online at:
<http://www.gnu.org/software/gdb/documentation/>.
For help, type "help".
Type "apropos word" to search for commands related to "word"...
Reading symbols from ./main...
(gdb) start
Temporary breakpoint 1 at 0x401111: file main.c, line 20.
Starting program: /home/others/Article-XX/main
warning: Error disabling address space randomization: Operation not permitted
Temporary breakpoint 1, main (argc=1, argv=0x7ffc9c096258) at main.c:20
20 unsigned int *puiPointer = NULL;
(gdb) list
15
16 /* main program entry */
17 int main(int argc, char **argv)
18 {
19 /* local variables */
20 unsigned int *puiPointer = NULL;
21
22 /* body program */
23
24 *puiPointer = 20;
(gdb) s
24 *puiPointer = 20;
(gdb) s
Program received signal SIGSEGV, Segmentation fault.
0x000000000040111d in main (argc=1, argv=0x7ffc9c096258) at main.c:24
24 *puiPointer = 20;
(gdb)
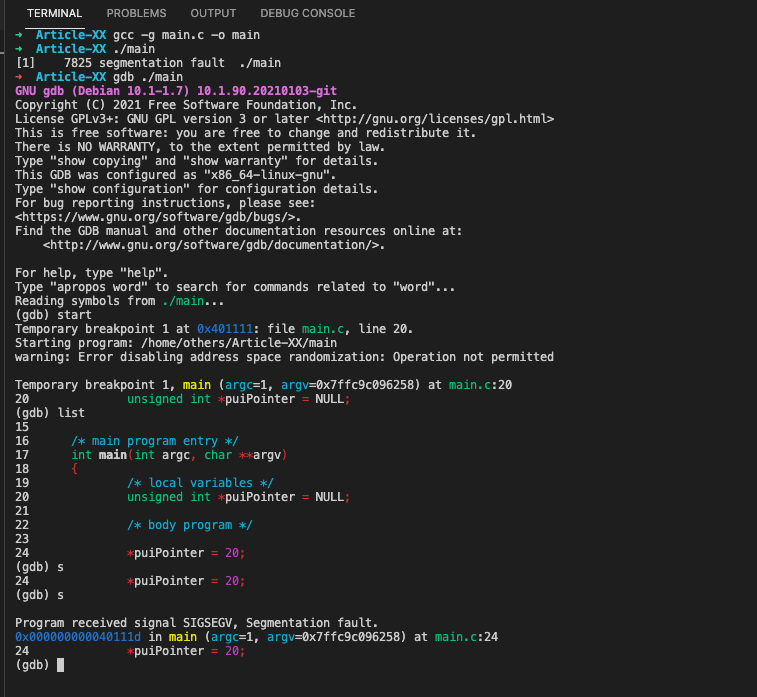
#b. Trying to access memory not initialized
Programs using uninitialized variables may crash when attempting to access uninitialized memory or may expose data stored in the uninitialized variables by writing to them. Also in the case when the program attempts to read or write to an area of memory not allocated with malloc(), calloc() or realloc().
An example of a simple segmentation fault is trying to read from a variable before it has been set:
/**
* @file main.c
* @author freecoder
* @brief this program allow to handle a segmentation fault error
*
* @version 1.0
* @date 8 Jan. 2022
*
* @copyright Copyright (c) 2022
*
*/
#include <stdio.h>
/* main program entry */
int main(int argc, char **argv)
{
/* local variables */
unsigned int *puiPointer;
/* body program */
*puiPointer = 20;
return 0;
}
In this case, the pointer puiPointer will be pointing to a random location in memory, so when the program attempts to read from it (by dereferencing *puiPointer), a segmentation fault will be triggered:
➜ Article-XX gcc -g main.c -o main
➜ Article-XX gdb ./main
GNU gdb (Debian 10.1-1.7) 10.1.90.20210103-git
Copyright (C) 2021 Free Software Foundation, Inc.
License GPLv3+: GNU GPL version 3 or later <http://gnu.org/licenses/gpl.html>
This is free software: you are free to change and redistribute it.
There is NO WARRANTY, to the extent permitted by law.
Type "show copying" and "show warranty" for details.
This GDB was configured as "x86_64-linux-gnu".
Type "show configuration" for configuration details.
For bug reporting instructions, please see:
<https://www.gnu.org/software/gdb/bugs/>.
Find the GDB manual and other documentation resources online at:
<http://www.gnu.org/software/gdb/documentation/>.
For help, type "help".
Type "apropos word" to search for commands related to "word"...
Reading symbols from ./main...
(gdb) start
Temporary breakpoint 1 at 0x401111: file main.c, line 24.
Starting program: /home/others/Article-XX/main
warning: Error disabling address space randomization: Operation not permitted
Temporary breakpoint 1, main (argc=1, argv=0x7fff6df4f038) at main.c:24
24 *puiPointer = 20;
(gdb) list
19 /* local variables */
20 unsigned int *puiPointer;
21
22 /* body program */
23
24 *puiPointer = 20;
25
26 return 0;
27 }
(gdb) s
Program received signal SIGSEGV, Segmentation fault.
0x0000000000401115 in main (argc=1, argv=0x7fff6df4f038) at main.c:24
24 *puiPointer = 20;
(gdb)
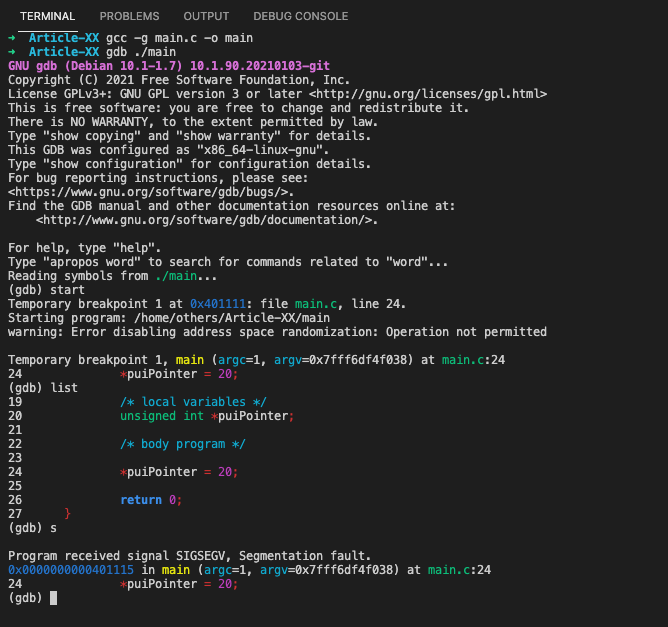
#c. Trying to access memory out of bounds for the program
In most situations, if a program attempts to access (read or write) memory outside of its boundaries, a segmentation fault error will occur. A code example of a simple segmentation fault error is below:
/**
* @file main.c
* @author freecoder
* @brief this program allow to handle a segmentation fault error
*
* @version 1.0
* @date 8 Jan. 2022
*
* @copyright Copyright (c) 2022
*
*/
#include <stdio.h>
/* main program entry */
int main(int argc, char **argv)
{
/* local variables */
unsigned int uiArray[20];
/* body program */
uiArray[5000] = 1;
return 0;
}
As shown bellow, the segmentation fault occurs after executing the out of bounds statement:
➜ Article-XX gcc -g main.c -o main
➜ Article-XX gdb ./main
GNU gdb (Debian 10.1-1.7) 10.1.90.20210103-git
Copyright (C) 2021 Free Software Foundation, Inc.
License GPLv3+: GNU GPL version 3 or later <http://gnu.org/licenses/gpl.html>
This is free software: you are free to change and redistribute it.
There is NO WARRANTY, to the extent permitted by law.
Type "show copying" and "show warranty" for details.
This GDB was configured as "x86_64-linux-gnu".
Type "show configuration" for configuration details.
For bug reporting instructions, please see:
<https://www.gnu.org/software/gdb/bugs/>.
Find the GDB manual and other documentation resources online at:
<http://www.gnu.org/software/gdb/documentation/>.
For help, type "help".
Type "apropos word" to search for commands related to "word"...
Reading symbols from ./main...
(gdb) start
Temporary breakpoint 1 at 0x401111: file main.c, line 23.
Starting program: /home/others/Article-XX/main
warning: Error disabling address space randomization: Operation not permitted
Temporary breakpoint 1, main (argc=1, argv=0x7ffdb68620f8) at main.c:23
23 uiArray[5000] = 1;
(gdb) list
18 {
19 /* local variables */
20 unsigned int uiArray[20];
21
22 /* body program */
23 uiArray[5000] = 1;
24
25 return 0;
26 }
(gdb) s
Program received signal SIGSEGV, Segmentation fault.
main (argc=1, argv=0x7ffdb68620f8) at main.c:23
23 uiArray[5000] = 1;
(gdb)
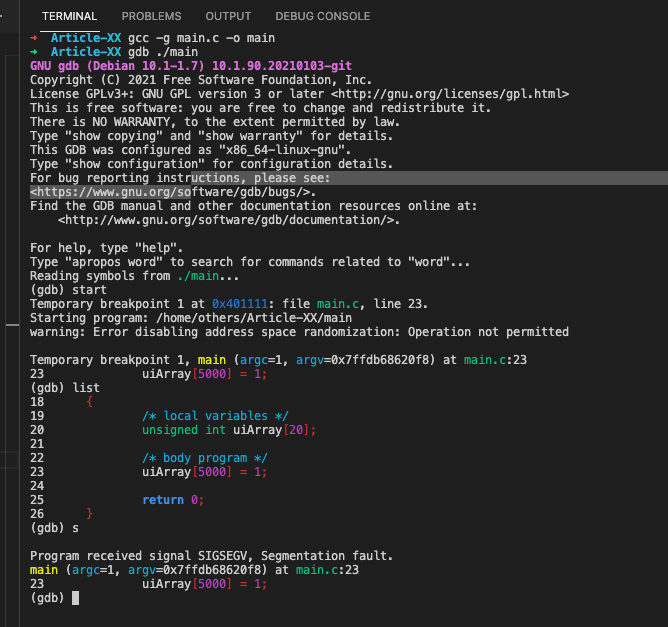
#d. Trying to modify string literals
/**
* @file main.c
* @author freecoder
* @brief this program allow to handle a segmentation fault error
*
* @version 1.0
* @date 8 Jan. 2022
*
* @copyright Copyright (c) 2022
*
*/
#include <stdio.h>
/* main program entry */
int main(int argc, char **argv)
{
/* local variables */
char* pucString = "Sample String 1";
/* body program */
pucString[14] = '2';
return 0;
}
As shown bellow, we got a segmentation error because the compiler put the string constant “Sample String 1” in read-only memory while trying to modify the contents of that memory which fails as a result:
➜ Article-XX gcc -g main.c -o main
➜ Article-XX gdb ./main
GNU gdb (Debian 10.1-1.7) 10.1.90.20210103-git
Copyright (C) 2021 Free Software Foundation, Inc.
License GPLv3+: GNU GPL version 3 or later <http://gnu.org/licenses/gpl.html>
This is free software: you are free to change and redistribute it.
There is NO WARRANTY, to the extent permitted by law.
Type "show copying" and "show warranty" for details.
This GDB was configured as "x86_64-linux-gnu".
Type "show configuration" for configuration details.
For bug reporting instructions, please see:
<https://www.gnu.org/software/gdb/bugs/>.
Find the GDB manual and other documentation resources online at:
<http://www.gnu.org/software/gdb/documentation/>.
For help, type "help".
Type "apropos word" to search for commands related to "word"...
Reading symbols from ./main...
(gdb) start
Temporary breakpoint 1 at 0x401111: file main.c, line 20.
Starting program: /home/others/Article-XX/main
warning: Error disabling address space randomization: Operation not permitted
Temporary breakpoint 1, main (argc=1, argv=0x7ffc2ea212e8) at main.c:20
20 char* pucString = "Sample String 1";
(gdb) list
15
16 /* main program entry */
17 int main(int argc, char **argv)
18 {
19 /* local variables */
20 char* pucString = "Sample String 1";
21
22 /* body program */
23 pucString[14] = '2';
24
(gdb) n
23 pucString[14] = '2';
(gdb)
Program received signal SIGSEGV, Segmentation fault.
main (argc=1, argv=0x7ffc2ea212e8) at main.c:23
23 pucString[14] = '2';
(gdb)
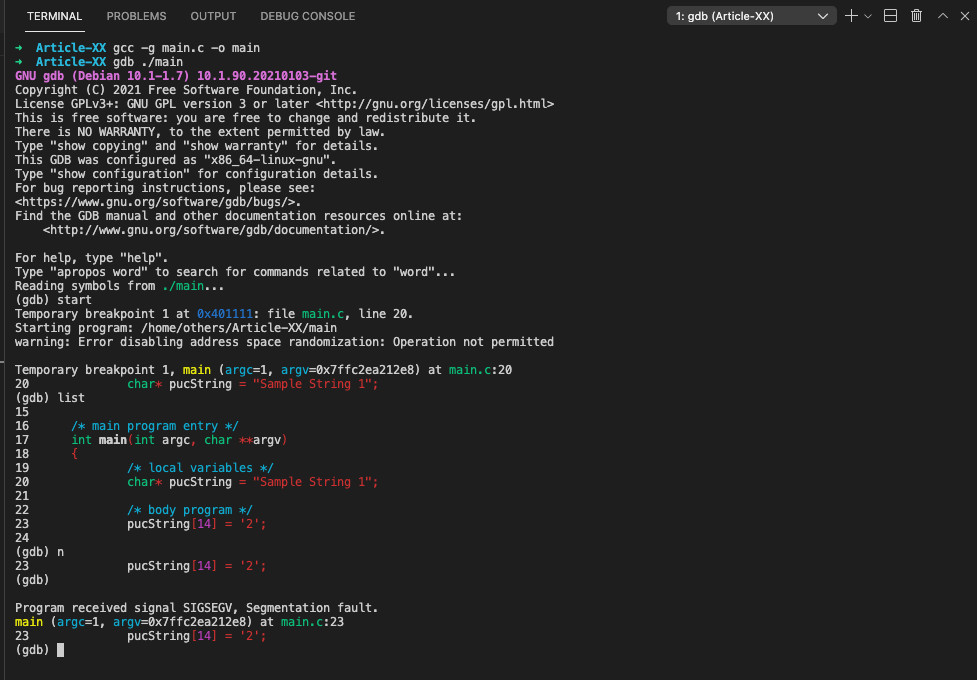
#e. Using variable's value as an address
A segmentation fault occurs when accidentally you are using a variable's value as an address as you can see through the code example bellow:
/**
* @file main.c
* @author freecoder
* @brief this program allow to handle a segmentation fault error
*
* @version 1.0
* @date 8 Jan. 2022
*
* @copyright Copyright (c) 2022
*
*/
#include <stdio.h>
/* main program entry */
int main(int argc, char **argv)
{
/* local variables */
int iVariable;
/* body program */
scanf("%d", iVariable);
return 0;
}
As shown in the terminal consol bellow, the segmentation occurs after the scans statement:
➜ Article-XX gcc -g main.c -o main
➜ Article-XX gdb ./main
GNU gdb (Debian 10.1-1.7) 10.1.90.20210103-git
Copyright (C) 2021 Free Software Foundation, Inc.
License GPLv3+: GNU GPL version 3 or later <http://gnu.org/licenses/gpl.html>
This is free software: you are free to change and redistribute it.
There is NO WARRANTY, to the extent permitted by law.
Type "show copying" and "show warranty" for details.
This GDB was configured as "x86_64-linux-gnu".
Type "show configuration" for configuration details.
For bug reporting instructions, please see:
<https://www.gnu.org/software/gdb/bugs/>.
Find the GDB manual and other documentation resources online at:
<http://www.gnu.org/software/gdb/documentation/>.
For help, type "help".
Type "apropos word" to search for commands related to "word"...
Reading symbols from ./main...
(gdb) start
Temporary breakpoint 1 at 0x401135: file main.c, line 23.
Starting program: /home/others/Article-XX/main
warning: Error disabling address space randomization: Operation not permitted
Temporary breakpoint 1, main (argc=1, argv=0x7fff418f9658) at main.c:23
23 scanf("%d", iVariable);
(gdb) list
18 {
19 /* local variables */
20 int iVariable;
21
22 /* body program */
23 scanf("%d", iVariable);
24
25 return 0;
26 }
(gdb) n
1
Program received signal SIGSEGV, Segmentation fault.
0x00007ff3e1d2201a in __vfscanf_internal (s=<optimized out>, format=<optimized out>, argptr=argptr@entry=0x7fff418f9460, mode_flags=mode_flags@entry=2)
at vfscanf-internal.c:1895
1895 vfscanf-internal.c: No such file or directory.
(gdb)
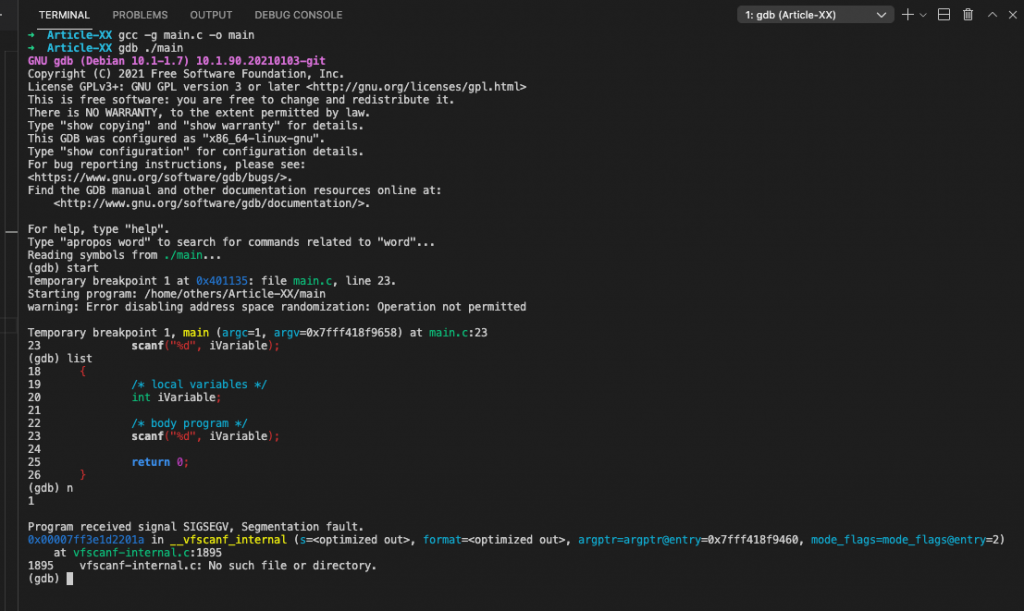
#f. Stack overflow
The segmentation fault error may occur if the call stack pointer exceeds the stack bound in case of an infinite recursive function call:
/**
* @file main.c
* @author freecoder
* @brief this program allow to handle a segmentation fault error
*
* @version 1.0
* @date 8 Jan. 2022
*
* @copyright Copyright (c) 2022
*
*/
#include <stdio.h>
/* main program entry */
int main(void)
{
/* local variables */
/* body program */
main();
return 0;
}
As shown bellow, the segmentation fault error happened, due to a stack oveflow after calling the main function:
➜ Article-XX gcc -g main.c -o main
➜ Article-XX gdb ./main
GNU gdb (Debian 10.1-1.7) 10.1.90.20210103-git
Copyright (C) 2021 Free Software Foundation, Inc.
License GPLv3+: GNU GPL version 3 or later <http://gnu.org/licenses/gpl.html>
This is free software: you are free to change and redistribute it.
There is NO WARRANTY, to the extent permitted by law.
Type "show copying" and "show warranty" for details.
This GDB was configured as "x86_64-linux-gnu".
Type "show configuration" for configuration details.
For bug reporting instructions, please see:
<https://www.gnu.org/software/gdb/bugs/>.
Find the GDB manual and other documentation resources online at:
<http://www.gnu.org/software/gdb/documentation/>.
For help, type "help".
Type "apropos word" to search for commands related to "word"...
Reading symbols from ./main...
(gdb) start
Temporary breakpoint 1 at 0x40110a: file main.c, line 22.
Starting program: /home/others/Article-XX/main
warning: Error disabling address space randomization: Operation not permitted
Temporary breakpoint 1, main () at main.c:22
22 main();
(gdb) list
17 int main(void)
18 {
19 /* local variables */
20
21 /* body program */
22 main();
23
24 return 0;
25 }
(gdb) n
Program received signal SIGSEGV, Segmentation fault.
main () at main.c:22
22 main();
(gdb)
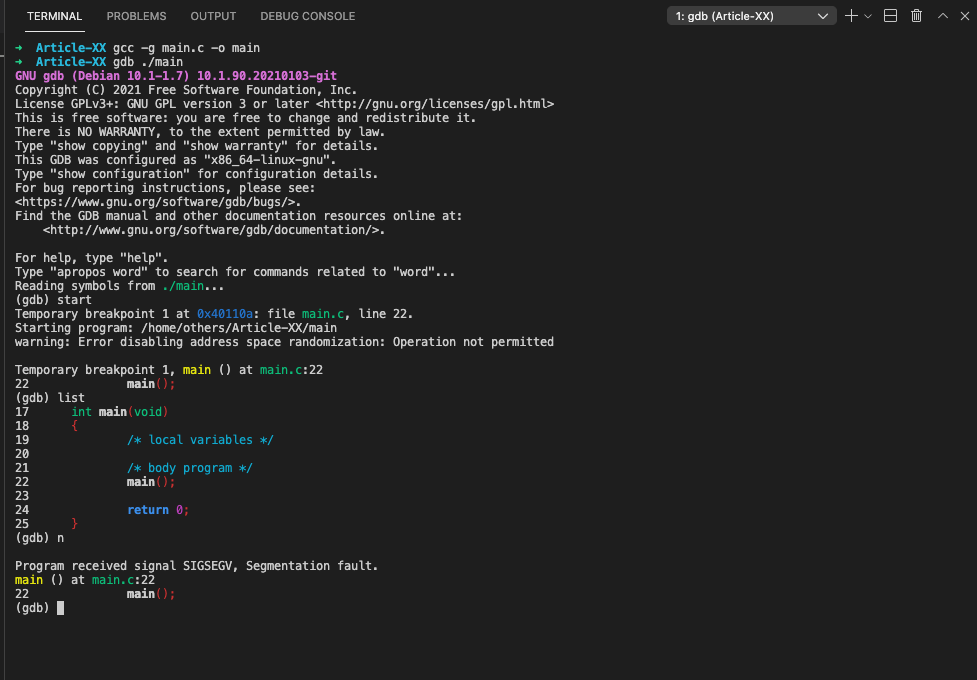
#2. How do you Fix Segmentation Faults?
Because segmentation faults are often associated with memory management issues or problematic pointer assignments, they can be fixed by making sure that the target application correctly handles these errors and does not attempt to read or write memory locations outside its own address space.
There are also certain procedures which you can follow in order to prevent and fix segmentation faults:
#a. How to Prevent Segmentation Faults?
Most segmentation faults occur due to memory access errors, so it's important to make sure that pointers used by an application always reference valid data areas.
- Check the reference of null memory.
- Testing the code with Valgrind or Electric Fence
- Assert() before dereferencing a suspective pointer, mainly a pointer embedded in a struct that is maintained in a container in a list or an array.
- Always remember to initialize pointers properly.
- Protect shared resources against concurrent access in multithreading by using a mutex or a semaphore.
- Use of free() routine
#b. How to Fix Segmentation Faults?
There are some tools that you can use in order to fix the segmentation faults:
Conclusion
A segmentation fault is generally caused by a programming bug that tries to access either non-existent or protected memory. It can also happen as a result of dividing an integer by zero (causing the program counter to be redirected to nowhere), accessing memory that is out of bounds at an address that does not contain valid data or code.
Finally, when enabled on some operating systems (and in some embedded programming environments), the processor may issue an exception if a memory address contains a non-mapped machine code instruction.
I hope this post has clarified what segmentation faults on the x86 architecture imply and how to avoid them. Do not forget to share the information on social networks if you believe it is useful for others. If you have any queries, please do not hesitate to leave a comment and subscribe to our newsletter. Best of luck with your coding and see you in the next article!