A common complaint from programmers is the lack of clear guidelines or some golden rules on what to do. Requirements are all over the place and it's impossible to know what to do in a given situation.
Programming has become so complex that no one person can read all the different tools, resources, and tutorials out there. This leaves programmers struggling with uncertainty and frustration.
In general, in the life cycle of a classic software, the program is written once but read, modified, or maintained several times in the days, months, years that follow.
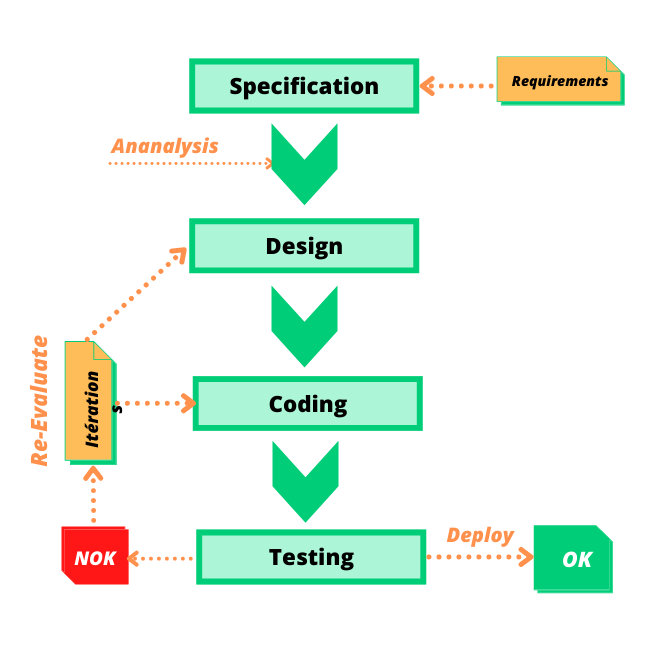
Hence the need to set up a certain software religion, a set of rules, and good practices to have a coherent, clear, and modular code.
These Guidelines will help you navigate through the sea of programming tools and requirements effortlessly. They're designed to be easy-to-read, concise, and will eliminate any ambiguity or confusion in your work. Read them now and see how much less of a struggle programming becomes!
#1. What are Guidelines for Formatting Code?
RULE_01: Use formatting without type underscores like Pascal or Camelcase for naming variables and functions.
RULE_02: Always add a space after each statement: if , switch, for, or while.
RULE_03: Use parentheses and braces in all your conditions and for reasons of clarity and readability of the code.
RULE_04: Use the symbol “/* */” for comments instead of “//” which can in some cases have side effects.
RULE_05: Comments must give added value they must be precise, clear, and short.
RULE_06: The line of code must not exceed 80 characters.
RULE_07: Use spaces for indentation and never use tabs for more clarity and visibility at the code level it is necessary to use indentation of 4 spaces.
#2. What are Rules for Better Robustness Code
RULE_01: Calling functions in conditions is forbidden so what to do is to call the function before, save its result in a variable and test it in the condition using this variable.
RULE_02: For more clarity and readability during mathematical processing and calculations, it is recommended to add a space before and after each operator: “+, -, *, =, /”.
RULE_03: Use a const type variable in your infinite loop because in unit testing it is very difficult or even impossible to test an infinite while(1) loop.
RULE_04: In the decision conditions type: “if, while, for” is forbidden to assign or perform processing operations on the variables.
RULE_05: In all conditional “if-else” blocks you must always put the “else” block even if it doesn’t contain any processing.
RULE_06: In the control conditions, you should never put more than four logical operations “&& or ||“ to not have a cyclomatic complexity greater than 20.
RULE_07: In switch case blocks, each block that contains a statement must end with a break.
RULE_08: Don’t forget also to end with the default case “default” in the conditional block of “switch case”.
RULE_09: In a “for” loop, it is strongly advised not to initialize other variables other than the counter of the loop.
RULE_10: Inside a “for” loop. Never change the value of its counter.
RULE_11: In “while” conditions testing an inequality is preferable to a permissive equality test only in the case of equality with a constant enum.
RULE_12: Local variables contain random data when they are created to avoid any problem related to this it is mandatory to initialize them before their use.
RULE_13: The variables except the const must never be reinitialized at the time of the declaration we first make the declaration than in another step their initialization.
RULE_14: Variables typed float should never be tested to their exact equality and it is better to add some tolerance (+-%) and that is for reasons of portability and robustness of the code.
RULE_15: Be very careful, the volatile keyword indicates that the content of the variable can change at any time in the code during its execution, which implicitly induces at the c compiler level to deactivate any optimization of the code on this resource.
RULE_16: Any numeric constant that has no functional meaning in the program should not be declared with a “#define”.
RULE_17: To avoid any surprises, it is strongly recommended to surround the “#define” constants with parentheses.
RULE_18: Must be very careful when using the sizeof instruction which can give unexpected results related to the non-alignment of certain data structures.
RULE_19: Always initialize the pointer to NULL before using it.
RULE_20: Global pointer should never be assigned to an address of a local variable or function.
RULE_21: In a “bit field” structure, it is necessary that:
- each element has a number of bits greater than 0
- the total number of bits is equal to the default type
RULE_22: In an “enum” statement, either:
- the first element is initialized only
- all elements are initialized
RULE_23: The use of the “continue” statement is prohibited.
RULE_24: The use of the “?” “is forbidden.
RULE_25: The use of the “goto” statement is prohibited.
RULE_26: The use of the break statement is prohibited outside the “switch-case”.
RULE_27: The use of the “macro” function is very limited to certain cases, otherwise prefer the definition of the classic function.
RULE_28: It is forbidden to have several “return” in different places of the function.
#3. What are Rules for Using Tools
RULE_01: For more coherence and homogeneity in your program, it is recommended to use template cartridges for the structure of the .c and .h files as well as cartridges for the declaration of functions.
RULE_02: It is strongly recommended to standardize collaborative tools such as development IDE, set up templates for notes or specification documents, design, and test.
RULE_03: It is strongly recommended to use a configuration and versioning manager like Git.
RULE_04: Using a tool like Doxygen allows both to have comments in the code a little more advanced and also to generate documentation of your code which can be easily communicated to third parties for example during code reviews.
RULE_05: Use a lint (PC-LINT or MISRA) to check and report warnings during compilation.
RULE_06: Use a tool for memory debugging, memory leak detection, and profiling like VALGRIND.
Conclusion
This document will be the best entry document for the new developer in addition to other specification or design input documents.
Also, having a uniform code allows the software team to implement tools for automating certain tedious tasks required during audits or software certifications: code/requirements traceability, code/tests, having code metrics, static code analysis.
All these coding rules described in this article are the result of several years of programming experience. Since I discovered and adapted these rules, I produce programs of better quality. If you don't really agree with any of these rules, you don't have to adopt them if you've found a better alternative let me know, I'm interested! 🙂