C programming is a difficult language to learn. Pointers are the hardest concept to pick up, which leaves frustrated programmers unable to create the programs they really want.
Not understanding pointers in code can lead to a myriad of errors and worst-case scenarios: memory leaks, runtime errors, and more.
This guide to pointers in C Programming will teach you what they are, how they work, when they're necessary, and when they're not so you can confidently move forward with your programming projects.
#1. What Is a Pointer
A pointer is a variable that stores the memory address of a variable or a function. It is often used to point to a particular location in memory where data or code resides. This concept was first introduced by Ken Thompson in his Turing Award lecture.
Pointers are the most confusing topic in C programming. However, they are also one of the most important topics to learn. Pointers are a type of data that stores an address or location of another variable.
Pointers allow you to access and manipulate data that is stored at any location in memory. With pointers, you can access any location in memory without having to know where it is located.
#2. How Pointers Work
Pointers are used in C programming language to allow programmers to pass memory addresses to functions. This is done by using an asterisk (*) symbol followed by the variable name.
Pointers have many uses in C programming such as passing parameters by reference (instead of by value), accessing objects that would otherwise not fit into memory or to avoid copying large data structures.
The use of pointers simplifies the process of accessing data and can also be used for creating dynamic data structures, like linked lists and trees, which are not possible without pointers.
#3. Why You Should Use Pointers in Your Code
Pointers are variables that store the address of another variable or data type in memory. They can be used to point to a single item or an array. The pointer itself is just a number, but it is interpreted differently depending on what type it points to.
Pointers are helpful because they allow programmers to move around large amounts of data without having to copy the entire thing each time they need to make changes. It also allows programmers to control how much memory their program uses by pointing only where they need more space instead of copying everything over and over again.
Indeed, pointing to the right memory location helps programmers to optimize their code and increase the speed of their programs.
Pointers are a fundamental part of programming and they are used to store the address of data in memory. Pointers can be used for optimization purposes by storing the address of data that is frequently accessed. This will increase the speed as it allows you to access this data without going through allocating and de-allocating memory.
#4. How do I Declare a Pointer?
A pointer is a data type that stores the memory address of another another variable, a constant, or a function.
The pointer is declared with an asterisk (*) followed by the type of data it will hold, and then the name of the variable it points to, as follows:
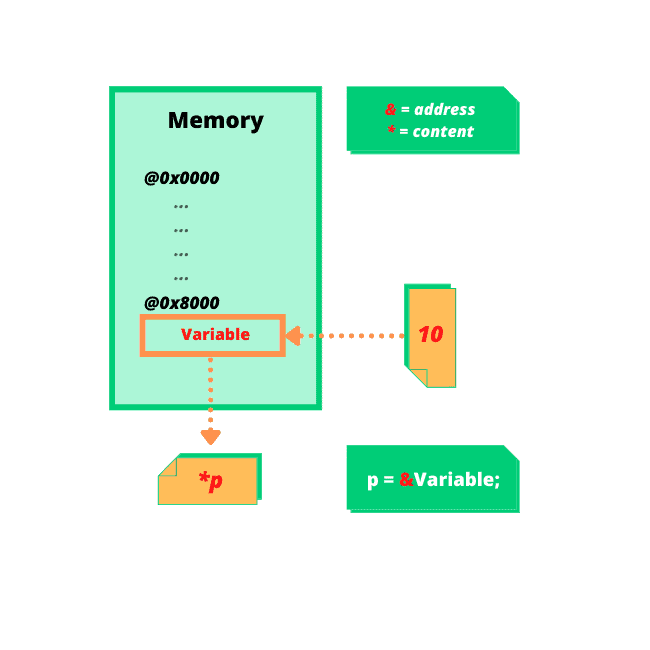
The following code snippet declares a pointer to an integer type:
int* pIntPointer;
pIntPointer = NULL;
The following code snippet declares and initializes a pointer to the square() function:
int (*pSquare)(int);
pSquare = square;
There are three ways to allocate dynamic memory pointer by using: malloc(), calloc() or realloc()
Name
malloc, free, calloc, realloc - allocate and free dynamic memory
Synopsis
#include <stdlib.h>
void *malloc(size_t size);
void free(void *ptr);
void *calloc(size_t nmemb, size_t size);
void *realloc(void *ptr, size_t size);
What are the Different Between these Memory Allocations Routines ?
- A malloc() is a function that allocates a block of memory. It does not initialize the allocated memory, and it is up to the programmer to ensure that the allocated memory is initialized properly.
- A calloc() is a function that allocates an array of space for an array of objects. It initializes each object in the allocated space to 0.
- A realloc() function changes the size of an existing block of memory, while keeping its contents intact. The old block must be freed before realloc can be used on it again, or else there will be a leak in your code.
#5. How can I Cast Values as Pointers?
The C language is a procedural language with strong typing, which means that all variables must be declared before use and cannot change their type during their lifetime. It also has automatic memory management, which means that the programmer does not need to allocate memory explicitly or free it when no longer needed.
Three ways of casting values as pointers are provided:
- Using a static cast: Converts a data type from one form to another.
- Using a const cast: Convert a value to a pointer.
- Using an explicit cast:
#6. How to Use Pointers Correctly
Pointers provide a powerful way to work with memory address of a variable, function or any other data type.
Pointers can be confusing and difficult to use correctly because they can cause errors that are difficult to debug. The following tips will help you avoid making mistakes with pointers:
- Avoid using pointers if you don't need to use them at all.
- Always initialize pointers before using them
- Don’t forget to release memory when you're done with it
- Use an asterisk (*) as a wildcard when declaring pointers
- Use NULL instead of 0 for pointer comparisons
- Use const references for passing pointers into functions
Conclusion
In conclusion, as we have seen in this article, pointers are one of the most important and powerful concepts in C programming.
On the other side, pointers are also important and play a key role in memory management, this huge process that involves allocating and de-allocating memory to meet the needs of the application.